로그인
resources/views/layouts/app.blade.php 파일을 열어서 로그인 버튼을 만듭니다.
<!doctype html>
<html lang="kr">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<link href="{{ asset('css/app.css') }}" rel="stylesheet">
<title>@yield('title', 'CRUD')</title>
</head>
<body>
@section('header')
<header class="w-2/3 mx-auto mt-16 text-right">
<a href="{{route('boards.index')}}" class="text-xl">게시판</a>
<a href="{{route('auth.register.index')}}" class="text-xl">회원가입</a>
<a href="{{route('auth.login.index')}}" class="text-xl">로그인</a>
</header>
@show
@section('section')
@show
</body>
</html>
resources/views/auth 폴더 안에 login.blade.php 파일을 만듭니다.
@extends('layouts.app')
@section('section')
<div class="w-2/3 mx-auto mt-16">
<form action="/auth/login" method="post" class="w-1/2 mx-auto">
@csrf
<p>
<label for="email" class="pr-2">이메일</label>
<input type="email" id="email" name="email"
class="outline-none border border-blue-400 rounded-lg pl-1 w-1/3">
</p>
<p class="mt-4">
<label for="password" class="pr-2">비밀번호</label>
<input type="password" id="password" name="password"
class="outline-none border border-blue-400 rounded-lg pl-1 w-1/3">
</p>
<p class="mt-8">
<input type="submit" value="로그인"
class="bg-blue-500 hover:bg-blue-700 px-4 py-1 text-lg text-white rounded-lg outline-none">
<input type="button" class="bg-red-500 hover:bg-red-700 px-4 py-1 ml-4 text-lg text-white rounded-lg outline-none"
value="취소" onclick="window.location.href ='{{route('home')}}'">
</p>
</form>
</div>
@stop
php artisan make:controller LoginController로 컨트롤러를 만들어줍니다.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class LoginController extends Controller
{
public function index(){
return view('auth.login');
}
public function login(Request $request){
$loginInfo = $request -> only(['email', 'password']);
if(auth() -> attempt($loginInfo)){
return redirect() -> route('home');
} else{
return redirect() -> route('auth.login.index');
}
}
public function logout(){
auth() -> logout();
return redirect() -> route('home');
}
}
$loginInfo에 이메일과 패스워드 정보를 저장하고
성공했을 시에는 메인화면으로, 실패했을 시에는 로그인화면으로 가게했습니다.
로그아웃도 메인화면으로 가게했습니다.
web.php에 라우트를 설정해줍니다.
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\BoardController;
use App\Http\Controllers\RegisterController;
use App\Http\Controllers\LoginController;
Route::get('/', function () {
return view('home');
}) -> name('home');
Route::get('auth/register', [RegisterController::class, 'index']) -> name('auth.register.index');
Route::post('auth/register', [RegisterController::class, 'store']) -> name('auth.register.store');
Route::get('auth/login', [LoginController::class, 'index']) -> name('auth.login.index');
Route::post('auth/login', [LoginController::class, 'login']) -> name('auth.login.attempt');
Route::post('auth/logout', [LoginController::class, 'logout']) -> name('auth.logout');
Route::get('boards', [BoardController::class, 'index']) -> name('boards.index');
Route::get('boards/create', [BoardController::class, 'create']) -> name('boards.create');
Route::post('boards', [BoardController::class, 'store']) -> name('boards.store');
Route::get('boards/{board}', [BoardController::class, 'show']) -> name('boards.show');
Route::get('boards/{board}/edit', [BoardController::class, 'edit']) -> name('boards.edit');
Route::put('boards/{board}', [BoardController::class, 'update']) -> name('boards.update');
이제 제대로 로그인이 됐는지 확인하기 위해 resources/views/layouts/app.blade.php 파일을 수정하겠습니다.
<!doctype html>
<html lang="kr">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<link href="{{ asset('css/app.css') }}" rel="stylesheet">
<title>@yield('title', 'CRUD')</title>
</head>
<body>
@section('header')
<header class="w-2/3 mx-auto mt-16 text-right">
<a href="{{route('boards.index')}}" class="text-xl">게시판</a>
@guest()
<a href="{{route('auth.register.index')}}" class="text-xl">회원가입</a>
<a href="{{route('auth.login.index')}}" class="text-xl">로그인</a>
@endguest
@auth()
<span class="text-xl text-blue-500">{{auth() -> user() -> name}}</span>
<form action="/auth/logout" method="post" class="inline-block">
@csrf
<a href="{{route('auth.logout')}}"><button class="text-xl">로그아웃</button></a>
</form>
@endauth
</header>
@show
@section('section')
@show
</body>
</html>
@guset와 @auth를 사용하면 미인증 사용자와 인증 사용자를 나눌 수 있습니다.
로그인하지 않을 경우에는 회원가입과 로그인을
로그인 했을 경우에는 사용자 이름과 로그아웃 버튼이 보이게 했습니다.
로그인 해서 실험 해보면 확인할 수 있습니다.
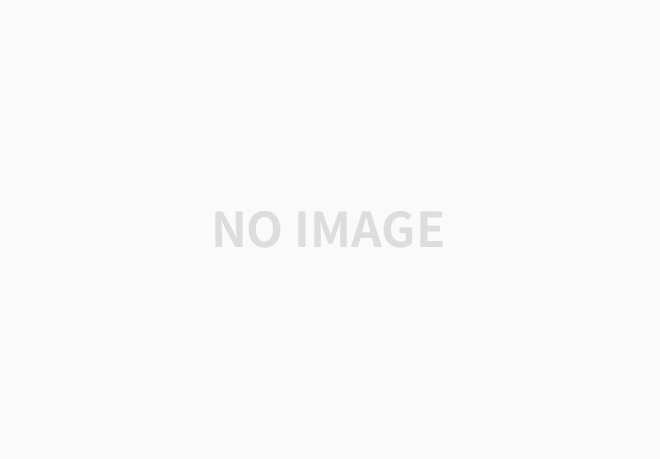
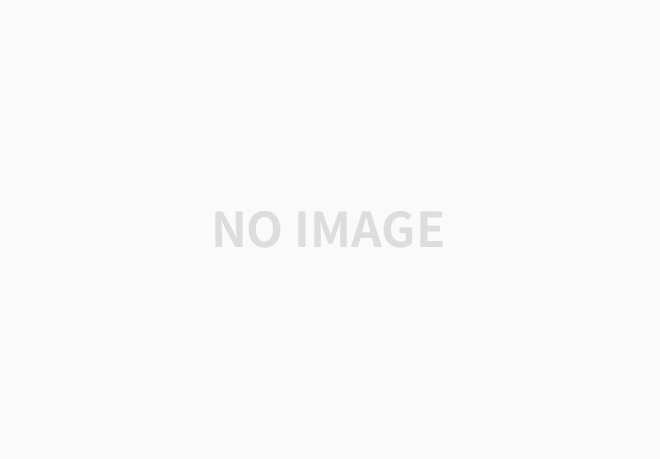
'IT > PHP' 카테고리의 다른 글
Laravel 8 - CRUD(9) (0) | 2021.01.08 |
---|---|
Laravel 8 - CURD(8) (0) | 2021.01.08 |
Laravel 8 - CRUD(6) (1) | 2021.01.07 |
Laravel 8 - CRUD(5) (1) | 2021.01.05 |
Laravel 8 - CRUD(4) (0) | 2021.01.05 |
댓글